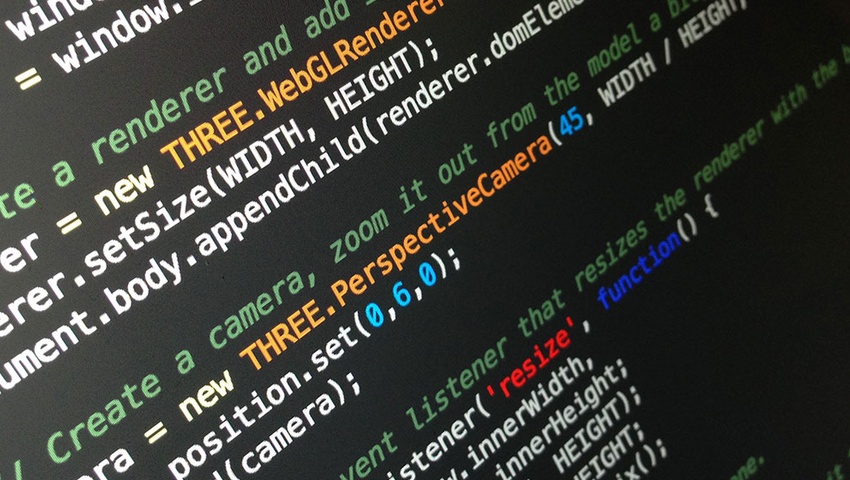
Example below will demonstrate how to add CodeMirror, a text editor implemented in Javascript, to the Django Textarea form widget by extending the class django.forms.Textarea
. Modified widget will be used as an example in Django Admin, but it can be easily ported to any Django form. CodeMirror editor implemented below is set to a HTML/Javascript mode. To support other languages/modes check the CodeMirror documentation.
CodeMirror is not the only one open source code editor, you might want to use. It's also worth, to check Ace, a nice looking Javascript based, code editor with similar functionality and programming languages syntax support. In my opinion, both editors are really good and similar to each other if comparing the functionality. The choice really depend on you.
Create project
Let's start by creating a new project and test app with Django 1.9. Inside the project/project/
directory create widgets.py
file, where our custom widget will be defined. After all, the tree structure should look like that:
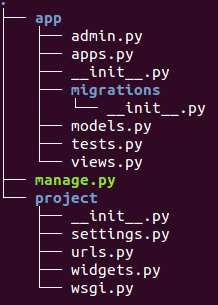
Create custom widget
We will use the HTML <textarea></textarea>
element as a code editor container. One of the Django widgets, the django.forms.Textarea
, already uses it. Let's extend this widget and add a necessary static files inside the Media
class. All listed static files will be included in the HTML document where the widget will be used.
Media
class works, have a look on the Django documentation here.
from django import forms
class HtmlEditor(forms.Textarea):
def __init__(self, *args, **kwargs):
super(HtmlEditor, self).__init__(*args, **kwargs)
self.attrs['class'] = 'html-editor'
class Media:
css = {
'all': (
'https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.9.0/codemirror.css',
)
}
js = (
'https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.9.0/codemirror.js',
'https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.9.0/mode/xml/xml.js',
'https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.9.0/mode/htmlmixed/htmlmixed.js',
'/static/codemirror-5.9/init.js'
)
The /static/codemirror-5.9/init.js
contains the initialization function for the CodeMirror editor.
(function(){
var $ = django.jQuery;
$(document).ready(function(){
$('textarea.html-editor').each(function(idx, el){
CodeMirror.fromTextArea(el, {
lineNumbers: true,
mode: 'htmlmixed'
});
});
});
})();
Add widget to Django admin
First let's specify some model we'll use to register in Django admin.
from django.db import models
class App(models.Model):
name = models.CharField(max_length=255)
code = models.TextField()
def __unicode__(self):
return self.name
Now we're ready to register the model in Django admin and use our custom widget for the code
model field of type django.db.models.TextField
.
from django import forms
from django.contrib import admin
from project.widgets import HtmlEditor
from .models import *
class AppAdminForm(forms.ModelForm):
model = App
class Meta:
fields = '__all__'
widgets = {
'code': HtmlEditor(attrs={'style': 'width: 90%; height: 100%;'}),
}
class AppAdmin(admin.ModelAdmin):
form = AppAdminForm
admin.site.register(App, AppAdmin)
Result
After this few steps we can use our custom code editor in Django admin.